配置 firebase
在创建了 firebase 项目以后,首先要将 Firebase Admin SDK 添加到服务器,然后生成配置 json 文件
firebase添加到服务器
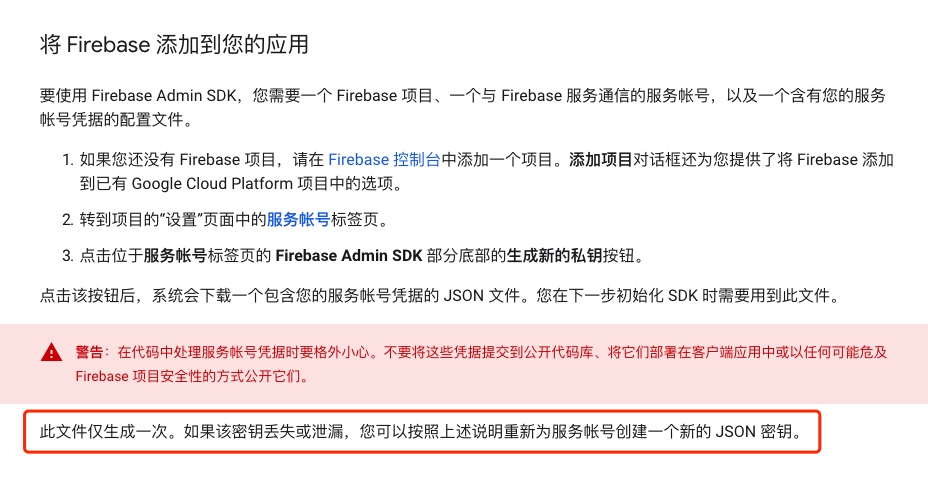
点击生成 JSON 文件以后,需备份保存,以免丢失
初始化 firebase SDK
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
|
'use strict';
const Service = require('egg').Service; const admin = require('firebase-admin');
const serviceAccount = require('../../config/firebase/wishes-firebase.json');
class FirebaseService extends Service { constructor(ctx) { super(ctx); admin.initializeApp({ credential: admin.credential.cert(serviceAccount), databaseURL: 'https://api-123123.firebaseio.com' }); this.admin = admin; } }
module.exports = FirebaseService;
|
消息推送
推送又分为特定设备推送,多台设备推送,向主题推送
具体的方法和数据结构
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
async sendMsg() { const { ctx, service } = this; const { title, body, userId } = ctx.request.body; const deviceToken = await service.device.getDeviceTokenByUserId(userId); const message = { notification: { title, body }, token: deviceToken }; await service.firebase.sendMsgToDevice(message).catch((err) => { this.app.logger.error(`fcm send msg err => ${err}`); throw new Error('firebase_error'); }); ctx.success(); }
async sendMsgToDevice(message) { await this.admin.messaging().send(message) .then((response) => { this.app.logger.debug(`Successfully sent message: ${response}`); }).catch((error) => { this.app.logger.error(`Error sending message: ${error}`); }); }
|
消息推送类型
使用 FCM,您可以向客户端发送两种类型的消息:
- 通知消息,有时被视为“显示消息”。此类消息由 FCM SDK 自动处理。
- 数据消息,由客户端应用处理。
message 的格式大概是这样
1 2 3 4 5 6 7 8 9 10 11 12 13
| { "message":{ "token":"bk3RNwTe3H0:CI2k_HHwgIpoDKCIZvvDMExUdFQ3P1...", "notification":{ "title":"Portugal vs. Denmark", "body":"great match!" }, "data" : { "Nick" : "Mario", "Room" : "PortugalVSDenmark" } } }
|
更多的格式请点击
使用 firebase 后台测试推送
firebase 后台
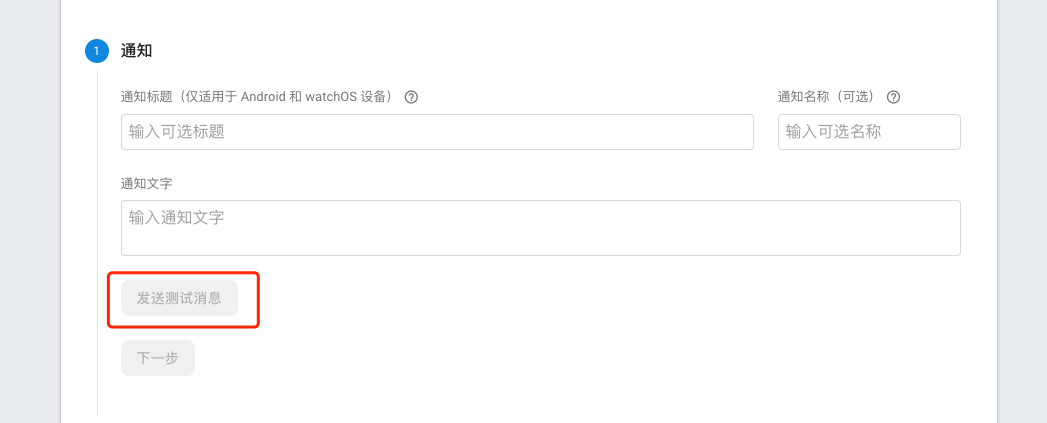
点击发送测试消息,输入设备的 deviceToken,就可以测试定向推送,测试通过了就可以拿该 deviceToken去调试代码了,优先确保 deviceToken 没有问题,手机应用能收到推送,再调试服务器代码.